` tag
-
-
-## HTML Attributes
-HTML elements can have attributes, **Attributes** provide additional information about the element, Attributes come in **name/value pairs** like **charset="utf-8"**
-
-
-
-
-
-**These are some basic HTML attributes**:
-- The **href** attribute
-HTML links are defined with the tag. The link address is specified in the href attribute:
-
-```html=
-This is a link
-```
-
-- The **src** Attribute
-HTML images are defined with the `
`tag. The filename of the image source is specified in the src attribute:
-
-```html=
-
-```
-
-- The **style** Attribute
-The *style* attribute is used to specify the styling of an element, like color, font, size etc.
-
-```html=
-This is a red paragraph.
-```
-
-- The **title** Attribute
-Here, a title attribute is added to the `` element. The value of the title attribute will be displayed as a tooltip when you mouse over the paragraph:
-
-```html=
-
- This is a paragraph.
-
-```
-
-
-For a full reference about HTML attributes visit:
-https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes
-
-
-## The HTML DOM
-When a web page is loaded, the browser creates a **D**ocument **O**bject **M**odel of the page. With the HTML DOM, **JavaScript** can access and change all the elements of an HTML document.
-
-**The HTML DOM model is constructed as a tree of Objects:**
-
-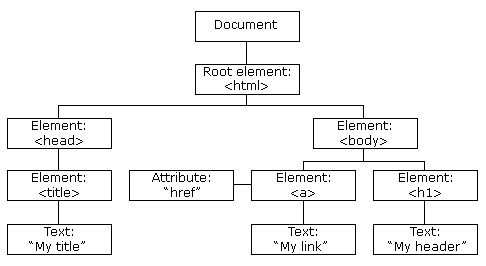
-
-From W3school https://www.w3schools.com/js/js_htmldom.asp
-
-We will learn more about the HTML DOM later in the course but its good to know what is it from now.
-
-
-## Basic HTML Structure For Web Page
-Here are the tags that pretty much any HTML page should have:
-
-- ``: This tag specifies the language you will write on the page. In this case, the language is HTML 5.
-
-- ``: This tag signals that from here on we are going to write in HTML code.
-
-- ``: This is where all the metadata for the page goes — stuff mostly meant for search engines and other computer programs.
-
-- ``: This is where the content of the page goes.
-
-
-
-
-
-This is how your average HTML page is structured visually.
-
-
-## Formatting Page Content with HTML
-We know now that we can add text to our HTML page using the `` but how can we format this text in HTML
-
-There are many ways of doing that but we will do it using some HTML elements:
-
-
-| Element | Usage |
-| ------- | ----- |
-| `` | Highlight important information|
-| `` | Similarly to bold, to highlight key text|
-| `` | To denote text |
-| `` | Usually used as image captions |
-| `` | Highlight the background of the text |
-| `` | To shrink the text |
-| `` | To place a horizontal line across the text |
-| `` | Used for links or text highlights |
-| `` | Typographical stylistic choice |
-| `` | Another typographical presentation style|
-
-To use or apply any of these formats on any text you need to just **wrap it** between the opening and closing tags
-
-```html=
-
- this word is normal but this
- word is bold
-
-```
-**The expected output**:
-
- this word is normal but this
- word is bold
-
-
-
----
-
-
-> In the following section, you will be introduced to some common HTML elements and we suggest that you try these elements on your own in a **CodePen**
-
-## Links
-Ever come across a link on a webpage that took you to another one. these links are HTML links made using the `` tag. In order for this tag to know where it should take the user when they click it, we have to give it an `href` attribute.
-
-**Example**
-
-```html=
-Click Me
-```
-**Can you guess where the link would take the user?** As you may have guessed when the user clicks this link he will be directed to **Google** page
-
-**The expected output**:
-
-Click Me
-
-
-## Images
-In today’s modern digital world, images are everything. The `
` tag has everything you need to display images on your site.
-
-In order for the image tag to display the image we need to provide it with the path to the image which can be a path for it on your device or a link from the web
-
-```html=
-
-```
-
-**The expected output** is an image displayed with no specific width or height so it should take all the space that it needs (its original size).
-
-
-
-
-## Lists
-We can create different types of lists in HTML and these two are the most common used:
-
-### Ordered List
-The first is an ``: This is an ordered list of contents. For example:
-
-1. An item
-2. Another item
-3. Another goes here.
-
-Inside the `` tag we list each item on the list inside `- ` `
` tags.
-
-**For example**:
-```html=
-
- - An item
- - Another item
- - Another goes here
-
-```
-**The expected output**
-
- - An item
- - Another item
- - Another goes here
-
-
-### Unordered List
-The second type of list that you may wish to include is an `` unordered list. This is better known as a bullet point list and contains no numbers.
-
-**An example of this**:
-
-```html=
-
- - This is
- - An Unordered
- - List
-
-```
-**The expected output**
-
- - This is
- - An Unordered
- - List
-
-
-## Tables
-When drawing a table we must open an element with the `` opening tag. Inside this tag, we structure the table using the table rows, ``, and cells, ``.
-
-**An example of an HTML table is as follows**:
-
-```html=
-
-
- Row 1 - Column 1 |
- Row 1 - Colunm 2 |
- Row 1 - Column 3 |
-
-
- Row 2 - Column 1 |
- Row 2 - Column 2 |
- Row 2 - Column 3 |
-
-
-```
-
-
-
- Row 1 - Column 1 |
- Row 1 - Colunm 2 |
- Row 1 - Column 3 |
-
-
- Row 2 - Column 1 |
- Row 2 - Column 2 |
- Row 2 - Column 3 |
-
-
-
-There are more additional elements that we can add to a table like the ` | ` which is for the table headings(A head/title for each column).
-
-```html=
-
-
- First Name |
- Last Name |
- Age |
-
-
- John |
- Doe |
- 18 |
-
-
-```
-
-The above HTML code above generates a table with two rows and three columns but the first row is for the table headings
-
-
-
- First Name |
- Last Name |
- Age |
-
-
- John |
- Doe |
- 18 |
-
-
-
-**Hint**: By default, the tables won't have any borders (in modern browsers) but some browsers add borders and some default styles to them.
-
-
-## Exercise
-
-Now that you know most of HTML elements that are used in most websites you should be able to make your own page with these elements so here is your task
-
-**Make An HTML Page About Yourself That Contains:**
-
-1. Your name which should be in an `` tag
-2. A paragraph about yourself using the `` tag and the text formatting tags **at least two** of them
-2. An image of you using the ` ` tag and specify a width and a height for it (150px/150px) and don't forget to add the **alt** attribute to it
-3. Make an ordered list about things that you like the most it could be about movies, songs, etc and make sure to give this list a heading using ` ` or `` heading elements
-4. Make an unordered list about goals you are looking to achieve sooner or later in your life, make sure to give it a heading like the previous list
-6. Each of these lists should include at least 4 items and one nested item under one of the items for example
- -
- Hello Song
-
-
-
-7. Make a table about your friends or any other people that has four columns and these columns has a heading (first name, last name, age, nickname) and fill it up with at least **three** data entries
-
-
-#### Congrats that's it! You have finished the workshop now look at the resources that we used in the workshop to learn more:
-
-https://www.w3schools.com/html/default.asp
-https://html.com/
-https://www.learn-html.org/
diff --git a/coursebook/session-01/README.md b/coursebook/session-01/README.md
index e69de29b..03360894 100644
--- a/coursebook/session-01/README.md
+++ b/coursebook/session-01/README.md
@@ -0,0 +1,16 @@
+# HTML INTRO
+
+### [Learning Outcomes](./learning-outcomes.md)
+
+### Schedule
+
+- 11:00 - 11:50 | [Intro To HTML](./intro-to-html.md)
+- 11:50 - 12:30 | [Elements And Attributes](./elements-and-attributes.md)
+
+ -**BREAK**-
+
+- 12:50 - 13:10 | [Elements And Attributes](./elements-and-attributes.md)
+- 13:10 - 14:00 | [Exercise](./exercise.md)
+
+
+### [Research Topics](./research-topics.md)
diff --git a/coursebook/session-01/elements-and-attributes.md b/coursebook/session-01/elements-and-attributes.md
new file mode 100644
index 00000000..8cbfeb42
--- /dev/null
+++ b/coursebook/session-01/elements-and-attributes.md
@@ -0,0 +1,184 @@
+## Formatting Page Content with HTML
+We know now that we can add text to our HTML page using the `` but how can we format this text in HTML
+
+There are many ways of doing that but we will do it using some HTML elements:
+
+
+| Element | Usage |
+| ------- | ----- |
+| `` | Highlight important information|
+| `` | Similarly to bold, to highlight key text|
+| `` | To denote text |
+| `` | Highlight the background of the text |
+| `` | To shrink the text |
+| `` | To place a horizontal line across the text |
+| `` | Used for links or text highlights |
+| `` | Typographical stylistic choice |
+| `` | Another typographical presentation style|
+
+[Check the difference between `` and ``](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/em)
+
+[Check the difference between `` and ``](https://www.seobility.net/en/wiki/Strong_and_Bold_Tags)
+
+To use or apply any of these formats on any text you need to just **wrap it** between the opening and closing tags
+
+```html
+
+ this word is normal but this
+ word is bold
+
+```
+**The expected output**:
+
+ this word is normal but this
+ word is bold
+
+
+
+---
+
+
+> In the following section, you will be introduced to some common HTML elements and we suggest that you try these elements on your own in a **CodePen**
+
+## Links
+Ever come across a link on a webpage that took you to another one. these links are HTML links made using the `` tag. In order for this tag to know where it should take the user when they click it, we have to give it an `href` attribute.
+
+**Example**
+
+```html
+Click Me
+```
+**Can you guess where the link would take the user?** As you may have guessed when the user clicks this link he will be directed to **Google** page
+
+**The expected output**:
+
+Click Me
+
+- [Explaining the noreferrer and noopener in the link tag](https://blog.templatetoaster.com/noopener-noreferrer/)
+
+## Images
+In today’s modern digital world, images are everything. The ` ` tag has everything you need to display images on your site.
+
+In order for the image tag to display the image we need to provide it with the path to the image which can be a path for it on your device or a link from the web
+
+```html
+
+```
+
+**The expected output** is an image displayed with no specific width or height so it should take all the space that it needs (its original size).
+
+
+
+
+## Lists
+We can create different types of lists in HTML and these two are the most common used:
+
+### Ordered List
+The first is an ``: This is an ordered list of contents. For example:
+
+1. An item
+2. Another item
+3. Another goes here.
+
+Inside the `` tag we list each item on the list inside `- ` `
` tags.
+
+**For example**:
+```html
+
+ - An item
+ - Another item
+ - Another goes here
+
+```
+**The expected output**
+
+ - An item
+ - Another item
+ - Another goes here
+
+
+### Unordered List
+The second type of list that you may wish to include is an `` unordered list. This is better known as a bullet point list and contains no numbers.
+
+**An example of this**:
+
+```html
+
+ - This is
+ - An Unordered
+ - List
+
+```
+**The expected output**
+
+ - This is
+ - An Unordered
+ - List
+
+
+## Tables
+When drawing a table we must open an element with the `` opening tag. Inside this tag, we structure the table using the table rows, ``, and cells, ``.
+
+**An example of an HTML table is as follows**:
+
+```html
+
+
+ Row 1 - Column 1 |
+ Row 1 - Colunm 2 |
+ Row 1 - Column 3 |
+
+
+ Row 2 - Column 1 |
+ Row 2 - Column 2 |
+ Row 2 - Column 3 |
+
+
+```
+
+
+
+ Row 1 - Column 1 |
+ Row 1 - Colunm 2 |
+ Row 1 - Column 3 |
+
+
+ Row 2 - Column 1 |
+ Row 2 - Column 2 |
+ Row 2 - Column 3 |
+
+
+
+There are more additional elements that we can add to a table like the ` | ` which is for the table headings(A head/title for each column).
+
+```html
+
+
+ First Name |
+ Last Name |
+ Age |
+
+
+ John |
+ Doe |
+ 18 |
+
+
+```
+
+The above HTML code above generates a table with two rows and three columns but the first row is for the table headings
+
+
+
+ First Name |
+ Last Name |
+ Age |
+
+
+ John |
+ Doe |
+ 18 |
+
+
+
+**Hint**: By default, the tables won't have any borders (in modern browsers) but some browsers add borders and some default styles to them.
diff --git a/coursebook/session-01/exercise.md b/coursebook/session-01/exercise.md
new file mode 100644
index 00000000..5b421c75
--- /dev/null
+++ b/coursebook/session-01/exercise.md
@@ -0,0 +1,20 @@
+## Exercise
+
+Now that you know most of HTML elements that are used in most websites you should be able to make your own page with these elements so here is your task
+
+**Make An HTML Page About Yourself That Contains:**
+
+1. Your name which should be in an `` tag
+2. A paragraph about yourself using the `` tag and the text formatting tags **at least two** of them
+2. An image of you using the ` ` tag and specify a width and a height for it (150px/150px) and don't forget to add the **alt** attribute to it
+3. Make an ordered list about things that you like the most it could be about movies, songs, etc and make sure to give this list a heading using ` ` or `` heading elements
+4. Make an unordered list about goals you are looking to achieve sooner or later in your life, make sure to give it a heading like the previous list
+6. Each of these lists should include at least 4 items and one nested item under one of the items for example
+ -
+ Hello Song
+
+
+
+7. Make a table about your friends or any other people that has four columns and these columns has a heading (first name, last name, age, nickname) and fill it up with at least **three** data entries
\ No newline at end of file
diff --git a/coursebook/session-01/intro-to-html.md b/coursebook/session-01/intro-to-html.md
new file mode 100644
index 00000000..d511be77
--- /dev/null
+++ b/coursebook/session-01/intro-to-html.md
@@ -0,0 +1,121 @@
+## Setup, Text Editors & Other Tools
+To go through the course and be able to apply what you learn in the workshops you need to have the following:
+
+- **A Browser**: Chrome (preferred), or Firefox or any modern browser
+- **A Text Editor**: VS Code (preferred), Atom, Sublime Text, Brackets or your preferred choice
+
+Make sure that you have these two installed before you continue.
+
+**Note**: Throughout the course we will use **Codepen** to try out/test some code snippets and these we will share with you so it would be great if you create a Codepen account now.
+
+
+## What is HTML?
+HTML stands for **H**yper **T**ext **M**arkup **L**anguage, HTML is the **standard markup** language for Web pages and HTML elements are the building blocks of HTML pages.
+
+### The History of HTML
+HTML was first created by Tim Berners-Lee, Robert Cailliau, and others starting in 1989.
+
+**Hypertext** means that the document contains links that allow the reader to jump to other places in the document or to another document altogether. The latest version is known as HTML5.
+
+A **Markup Language** is a way that computers speak to each other to control how text is processed and presented. To do this HTML uses two things: tags and attributes
+
+Read more: https://html.com/#ixzz6DGtsrDHr
+
+
+## HTML Elements
+As we mentioned in the previous paragraph, **HTML Elements** are the building blocks of a webpage. An HTML element is a **start tag** and an **end tag** with content in between.
+
+```html
+ Hello World
+```
+
+This is an example of an HTML element which represents a big heading as you can see the starter tag is `` and the end tag is `` and the content `hello world` goes between them.
+
+
+
+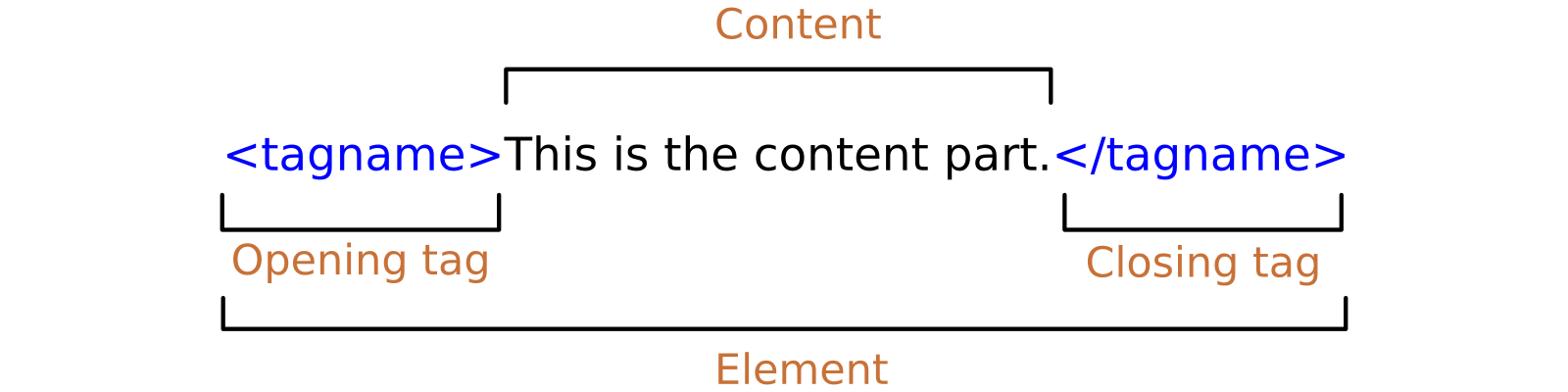
+
+
+**The basic elements of an HTML page are**:
+
+- A text header, denoted using the ``, ``, ``, ``, ``, `` tags.
+- A paragraph, denoted using the `` tag.
+- A horizontal ruler, denoted using the ` ` tag.
+- A link, denoted using the `` (anchor) tag.
+- A list, denoted using the `` (unordered list), `` (ordered list) and `- ` (list element) tags.
+- An image, denoted using the `
` tag
+- A divider, denoted using the `` tag
+- A text span, denoted using the ` ` tag
+
+
+## HTML Attributes
+HTML elements can have attributes, **Attributes** provide additional information about the element, Attributes come in **name/value pairs** like **lang="ar"**
+
+
+
+
+
+**These are some basic HTML attributes**:
+- The **href** attribute
+HTML links are defined with the tag. The link address is specified in the href attribute:
+
+```html
+This is a link
+```
+
+- The **src** Attribute
+HTML images are defined with the ` `tag. The filename of the image source is specified in the src attribute:
+
+```html
+
+```
+
+- The **style** Attribute
+The *style* attribute is used to specify the styling of an element, like color, font, size etc.
+
+```html
+This is a red paragraph.
+```
+
+- The **title** Attribute
+Here, a title attribute is added to the `` element. The value of the title attribute will be displayed as a tooltip when you mouse over the paragraph:
+
+```html
+
+ This is a paragraph.
+
+```
+
+
+For a full reference about HTML attributes visit:
+https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes
+
+
+## The HTML DOM
+When a web page is loaded, the browser creates a **D**ocument **O**bject **M**odel of the page. With the HTML DOM, **JavaScript** can access and change all the elements of an HTML document.
+
+**The HTML DOM model is constructed as a tree of Objects:**
+
+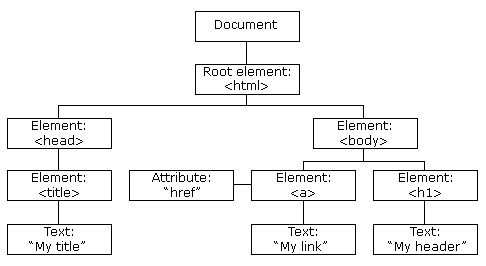
+
+From W3school https://www.w3schools.com/js/js_htmldom.asp
+
+We will learn more about the HTML DOM later in the course but its good to know what is it from now.
+
+
+## Basic HTML Structure For Web Page
+Here are the tags that pretty much any HTML page should have:
+
+- ``: This tag specifies the language you will write on the page. In this case, the language is HTML 5.
+
+- ``: This tag signals that from here on we are going to write in HTML code.
+
+- ``: This is where all the metadata for the page goes — stuff mostly meant for search engines and other computer programs.
+
+- ``: This is where the content of the page goes.
+
+
+
+
+
+This is how your average HTML page is structured visually.
diff --git a/coursebook/session-01/learning-outcomes.md b/coursebook/session-01/learning-outcomes.md
new file mode 100644
index 00000000..f82f711a
--- /dev/null
+++ b/coursebook/session-01/learning-outcomes.md
@@ -0,0 +1,11 @@
+# Session 01: HTML-01
+Welcome to the first session about HTML, this is going to be a great guide to get you started with HTML which is what every web page consists of.
+
+**Learning Outcomes**
+- Picking a code editor and get familiar with other tools we will be using in the course
+- Basic understanding of HTML: DOM, Element, Attributes
+- Understand the basic structure for a web page
+- Formatting Page Content with HTML
+- Get to know and use some HTML elements: Links, Lists, Tables and more
+
+**Note**: At the end of the workshop we will have 15 mins for questions so prepare questions for us.
diff --git a/coursebook/session-01/research-topics.md b/coursebook/session-01/research-topics.md
new file mode 100644
index 00000000..157fdfca
--- /dev/null
+++ b/coursebook/session-01/research-topics.md
@@ -0,0 +1,8 @@
+## Research Topics
+These topics will help you understand today's workshop better and be well prepared for the next workshop
+
+- Images in HTML
+- Links in Web pages
+- Semantic HTML
+
+Good luck! and I hope these were helpful to you
diff --git a/coursebook/session-01/resources.md b/coursebook/session-01/resources.md
new file mode 100644
index 00000000..c69a8353
--- /dev/null
+++ b/coursebook/session-01/resources.md
@@ -0,0 +1,7 @@
+#### Congrats that's it! You have finished the workshop now look at the resources that we used in the workshop to learn more:
+
+https://www.w3schools.com/html/default.asp
+
+https://html.com/
+
+https://www.learn-html.org/
| |