-
Notifications
You must be signed in to change notification settings - Fork 4
Design Patterns
We used Model-View-Controller (MVC) design pattern in our game development. It follows that each of the components has its own pattern as shown below. For instance, PauseGameController (controller) doesn't directly update the GameWindow (view) since it this done by the GameLoopInteractorReference (the model).
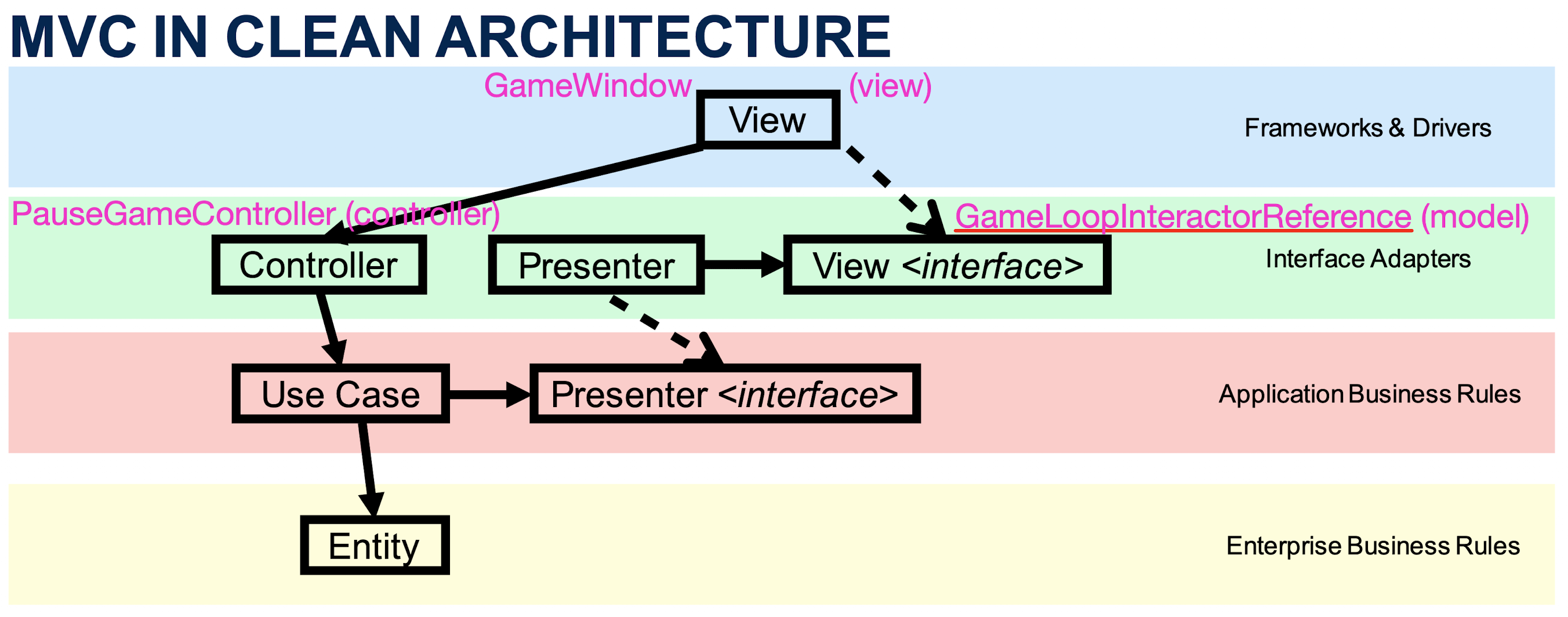
The Observer Design Patten represents the logic of our application (Model). Through this pattern, the Model is able to interact with the views and controllers without knowing anything about them. The GameLoopInteractorReference interface defines a mechanism that notifies the GameWindow about any events that happen to the object they’re observing, in this case, the paused screen. This pattern makes the interaction between all classes loosely coupled.
The strategy pattern decouples user inputs from the game's logic (Model) and interfaces (View). This pattern lets us define a family of algorithms, put each of them into a separate class, and make their objects interchangeable. For example, PauseGameController takes the current game state and the game manager reference into separate classes making each of them interchangeable. Therefore, it separates the implementation of the class from the performance of the algorithms.
The Composite Design Pattern represents an application's view (the game window & user input). This pattern lets us compose objects into tree structures and then work with these structures as if they were individual objects. The GameWindow class wraps all the different components on the screen to be controlled as one view, hence providing a unified access point for all views of the model. The design composes more similar objects so that they can be manipulated as one object
Below is a summary of the designs discussed above